iOS SDK Setup
https://git.messangi.com/messangi/messangi-ios-sdk
Requirements
To use the messaging SDK, the following is required:
A registered Apple Developer account
Follow the installation steps:
Configure CocoaPod
Configure XCode Project
Open the AppDelegate file of your project
Installation
Step 1: Configure CocoaPod
Open Terminal and start CocoaPod on the project that you are working on.
cd /YourProject pod init nano Podfile
Place the “MessagingSDK” dependency
pod 'MessagingSDK'
Run the installation or update command as appropriate
pod install # or pod update
Open the workspace created by cocoapod for your project
open YourProject.xcworkspace
Step 2: Configure XCode Project
Select your project and go to the Signatures and Capabilities tab.
Select a valid device to configure the following capabilities:
Add Push Notifications
Add Background modes and enable the following:
Location updates
Add Keychain Sharing and set a keychain group name
com.messaging.MessagingSDK
Step 3: Obtain Messaging-Info.plist file
Obtain the configuration file Messaging-Info.plist from the Mobile Messaging Platform. This is found in the Preferences section under the Push tab after completing the initial App Integration setup.
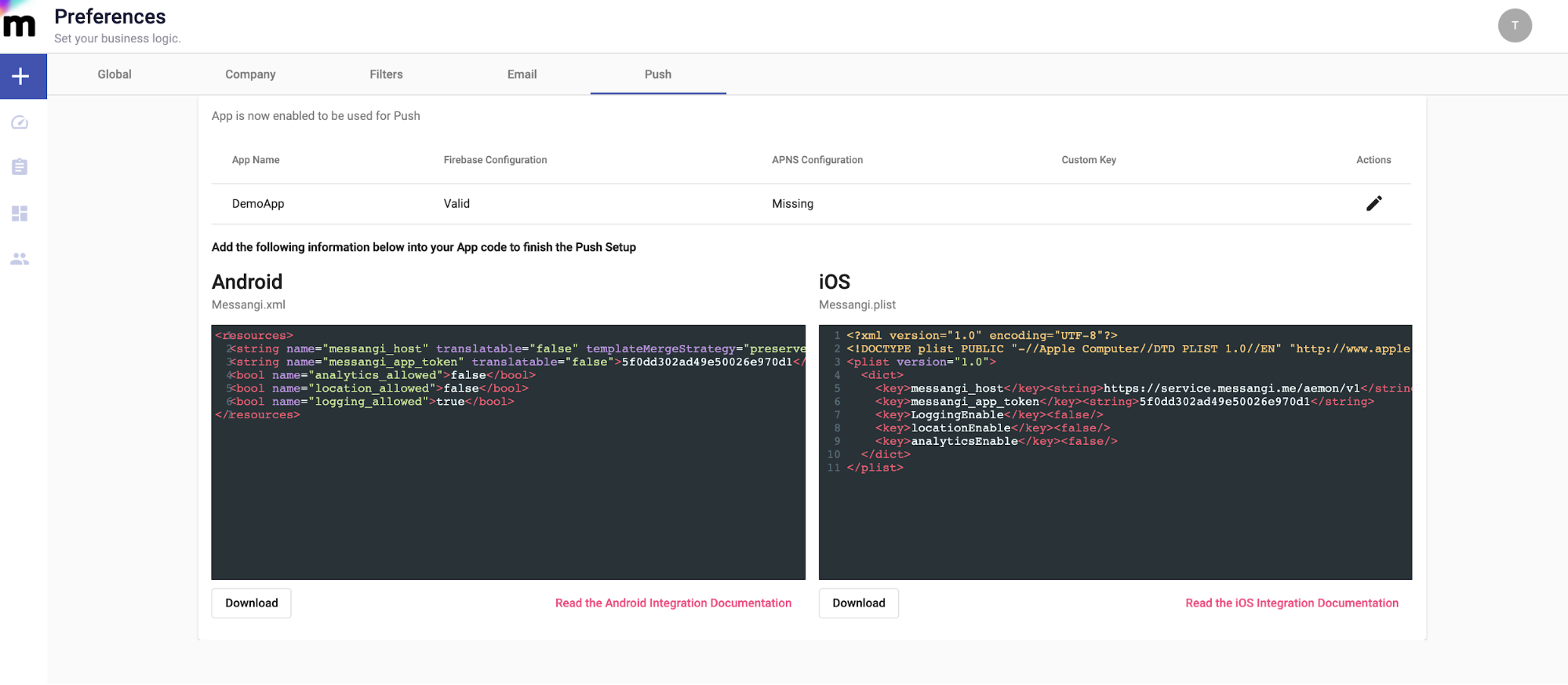
Example of Messaging-Info.plist file:
<!DOCTYPE plist PUBLIC "-//Apple Computer//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd"> <plist version="1.0"> <dict> <key>messagingHost</key><string>YourHost</string> <key>messagingAppToken</key><string>YourAppToken</string> <key>loggingEnable</key><false/> <key>locationEnable</key><false/> <key>analyticsEnable</key><false/> </dict> </plist>
Once you have downloaded the requiredMessaging-Info.plistfile, enter the following values:
messagingHost string: it must contain the URL and host version of messaging.
messagingAppToken string:access code granted by messaging.
loggingEnable boolean: variable to activate or deactivate the logs from the SDK
locationEnableboolean: variable to activate or deactivate Location use in the SDK.
analyticsEnable boolean: variable to activate or deactivate the Analytics use in the SDK.
Step 4: Open the AppDelegate project file
Select your project and go to the Signatures and Capabilities tab. Select a valid device to configure the following capabilities:
Implicit Implementation
Open your AppDelegateimport MessagingSDK module and replace the parent classUIResponder and implementationUIApplicationDelegate withMessagingAppDelegate
import MessagingSDK @UIApplicationMain class AppDelegate: MessagingAppDelegate { // you can leave this empty if you're using a storyboard. }
Note
You can overwrite theMessagingAppDelegate methods in your AppDelegate, but remember to invoke the parent via super.
@UIApplicationMain class AppDelegate: MessagingAppDelegate { override func application(_ application: UIApplication, didFinishLaunchingWithOptions // your code return super.application(application, didFinishLaunchingWithOptions: launchOptions) } }
Explicit Implementation
If you cannot do without the current parent class of your project, place the following invocations in the following methods of your AppDelegate.
In the applicationDidFinishLaunchingWithOptions method place MessagingImplementation.initialize(), in this manner:
override func application(_ application: UIApplication, didFinishLaunchingWithOptions la MessagingImplementation.initialize() // your code... return true }
In the applicationDidRegisterForRemoteNotificationsWithDeviceToken method, place MessagingImplementation.indicate(pushToken: deviceToken), in this manner:
override func application(_ application: UIApplication, didRegisterForRemoteNotification MessagingImplementation.indicate(pushToken: deviceToken) } override func applicationWillEnterForeground(_ application: UIApplication) { MessagingImplementation.checkChanges() }
Also in method application(:didReceiveRemoteNotification:fetchCompletionHandler) placeMessagingImplementation.didReceiveRemoteNotification(:fetchCompletionHandler), this way:
func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [A MessagingngImplementation.didReceiveRemoteNotification(userInfo, fetchCompletionHand }
Finally you can implement the MessagingNotificationDelegate interface in your AppDelegate class to manage what happens when a notification arrives.
... class AppDelegate: ..., MessagingNotificationDelegate { ... } ...
Usage
To make use of the functionalities that Messaging SDK offers, the Messaging class is available, to obtain an instance of this class you can do.
let messaging = Messaging.shared
By doing this you have access to the following:
Messaging.shared.requestPermissionForPushNotifications() Messaging.shared.requestDevice(forceCallToService:) Messaging.shared.observe\EventName/ Messaging.shared.removeObserve\EventName/
Deep Linking
MessagingSDK allows for Push Notifications to contain a link to a section inside of the Application or to a Web Page link.
For this we have 3 modalities:
Explicit behavior using Payload
URL Schema
Universal Links
Payload
To use this modality, indicate the behavior that will occur once the Push Notification is received, in the following way:
... import MessagingSDK @UIApplicationMain class AppDelegate: MessagingAppDelegate or ... MessagingUserNotificationDelegate { ... override func messagingReceivedDeepLink(_ notification: MessagingNotification) { let storyboard = UIStoryboard.init(name: "Main", bundle: nil) if let root = window?.rootViewController as? UINavigationController, let detail = storyboard.instantiateViewController(withIdentifier: "detailVCI detail.notification = notification root.pushViewController(detail, animated: true) } } ... }
In this example, we ask if the root view of the application is a navigation controller and we look in the Main.storyboard file for a view controller that has the detailVCId identifier, if both premises are correct.
You pass the notification parameter to make use of the data within the definition of my class NotificationDetailViewController and you place this screen for the user to visualize it.
MessagingReceivedDeepLink is a function that is invoked when a notification of type deeplink is received and is found in MessagingAppDelegate or MessagingUserNotificationDelegate, the idea is to define what to do with the overwriting of this method.
Note: For the execution of this method, the user is required to interact with the Push Notification, so it must be shown on the screen with themessagingReceivedNotificationfunction:
override func messagingReceivedNotification(_ notification: MessagingNotification, w -> UNNotificationPresentationOptions { if notification.deepLink { return [.alert, .sound] } } ... }
In this example, if a Deep Link Notification is received, it is displayed on the screen.
URL Schema
A linking Scheme allows you to associate a link with your Application.
This approach uses the linking scheme to determine the Application linked to that Scheme.
Once configured, you will have a link that will open your Application and process this link as needed.
To associate a URL Scheme to your Application, open your project settings, enter into the Info tab, and click on the URL types section.
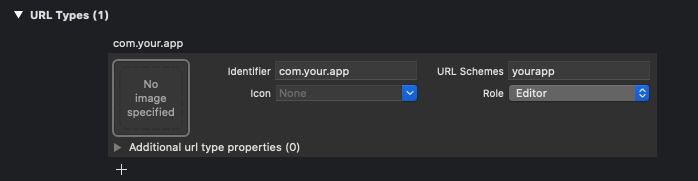
This is an example of a linking scheme:
yourapp://sectionapp?param1=value1&...¶mN=valueN
You can use this link in a Push Notification or Website. Indicate that it is a Deeplink Push Notification and enter the link in an attribute called Schema.
As an option, you may put other parameters of interest since these will be added to the URL when it is being processed.
Example: Website
... <a href="yourapp://sectionapp?param1=value1&...¶mN=valueN">See more</a> ...
Example: Push Notification Data
... "data": { "deepLink": true, "schema": "yourapp://sectionapp?param1=value1&...¶mN=valueN", "another1": "value1", ... "anotherX": "valueX" } ...
The following method is used to indicate the behavior that will be done to process the URL:
func application(_ app: UIApplication, open url: URL, options: [UIApplication.OpenURLOpt // your code // example: open your view controller using the data url. return true }
Now all the information received by the URL can be extracted from the URL object, or if you wish, use another structure like NSURLComponents to support the methods you have and obtain the path or the query parameters
In this example, we use NSURLComponents to extract the information from the URL and place it in a dictionary:
var urlData:[String: Any?] = [:] urlData["url.absoluteString"] = url.absoluteString if let components = NSURLComponents(url: url, resolvingAgainstBaseURL: true){
Universal Link
Examples
Full Example
You are in the following repository, you should just clone the repository, have the library at the same level of the project and open the file FullDemonstrationMessagingSDK.xcworkspace, I leave some screenshots of the application.
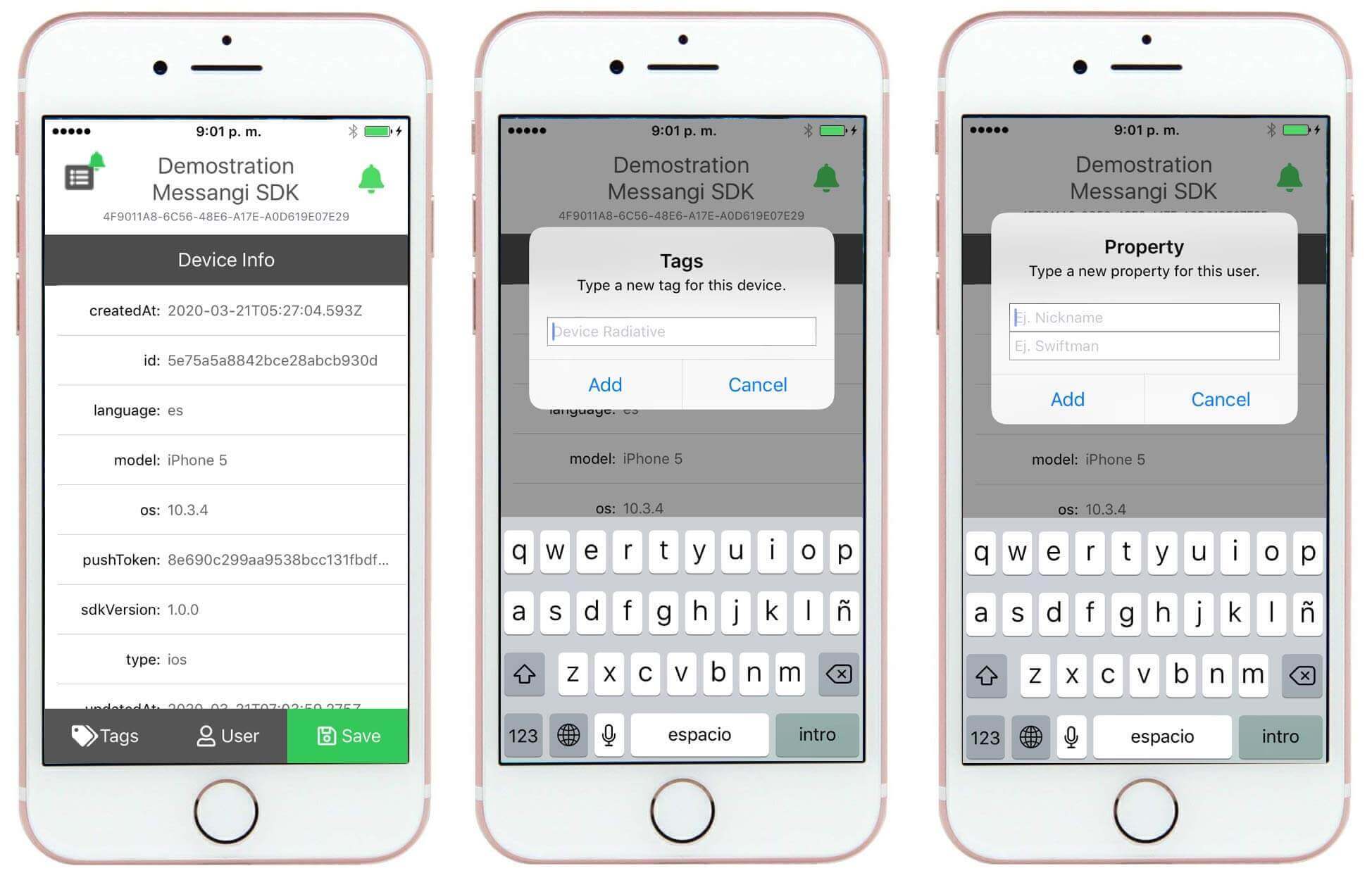
Example - Getting MessagingDevice
... import MessagingSDK class ExampleViewController: UIViewController { ... private var device: MessagingDevice? override func viewDidLoad() { ... _ = Messaging.shared.observeDeviceFetchResponse(self, do: #selector(workWithDevi ... } func onAnyEventToGetDevice(){ Messaging.shared.requestDevice() } @objc func workWithDevice( _ notification: Notification){ if let device = notification.object as? MessagingDevice { // MessagingDevice availability self.device = device } } override func viewDidDisappear(_ animated: Bool) { ... _ = Messaging.shared.removeObserveDeviceFetchResponse(self) ... } } ... import MessagingSDK class ExampleViewController: UIViewController { ... private var user: MessagingUser? override func viewDidLoad() { ... _ = Messaging.shared.observeUserFetchResponse(self, do: #selector(workWithUser(_ ... } func onAnyEventToGetUser(){ // Need MessagingDevice device?.requestUser() } @objc func workWithUser( _ notification: Notification){ if let user = notification.object as? MessagingUser { // MessagingUser availability self.user = user } } override func viewDidDisappear(_ animated: Bool) { ... _ = Messangi.shared.removeObserveUserFetchResponse(self) ... } }
Example - Getting a MessagingNotification (willPresent)
Process all the Notifications that arrive at the device, for both background and foreground. This is obtained regardless of whether the user interacts with the device or not.
... import MessagingSDK @UIApplicationMain class AppDelegate: MessagingAppDelegate or ... MessagingUserNotificationDelegate { override func messagingReceivedNotification(_ notification: MessagingNotification) - ... // your code // notification.actionIdentifier // has your custom action identifier } }
Another way to process Notifications is through an observer:
... import MessagingSDK class ExampleViewController: UIViewController { ... private var ReceivedNotification: MessagingNotification? override func viewDidLoad() { ... _ = Messaging.shared.observeReceivedNotification(self, do: #selector(workWithUse ... } // Unlike the previous examples, this case does not require a request for notificati @objc func workWithNotification( _ notification: Notification){ if let messagingNotification = notification.object as? MessagingNotification { // MessagingNotification availability self.ReceivedNotification = messagingNotification ... _ = Messangi.shared.removeObserveReceivedNotification(self) ... } }
Example - To control the interaction of the notification (didReceive)
This method will only run when the user clicks on the received Notification on their device.
... import MessagingSDK @UIApplicationMain class AppDelegate: MessagingAppDelegate or ... MessagingUserNotificationDelegate { override func messagingInteractionNotification(_ notification: MessagingNotification ... // your code // notification.actionIdentifier // has your custom action identifier } }